This new capability allows developers to define interactions with basemap and custom features in the style with simpler code, saving them hours of time building and maintaining map interactions. Interactions can now also be built into features in the Mapbox Standard 3D style.
Simplified system for building interactions
Heading
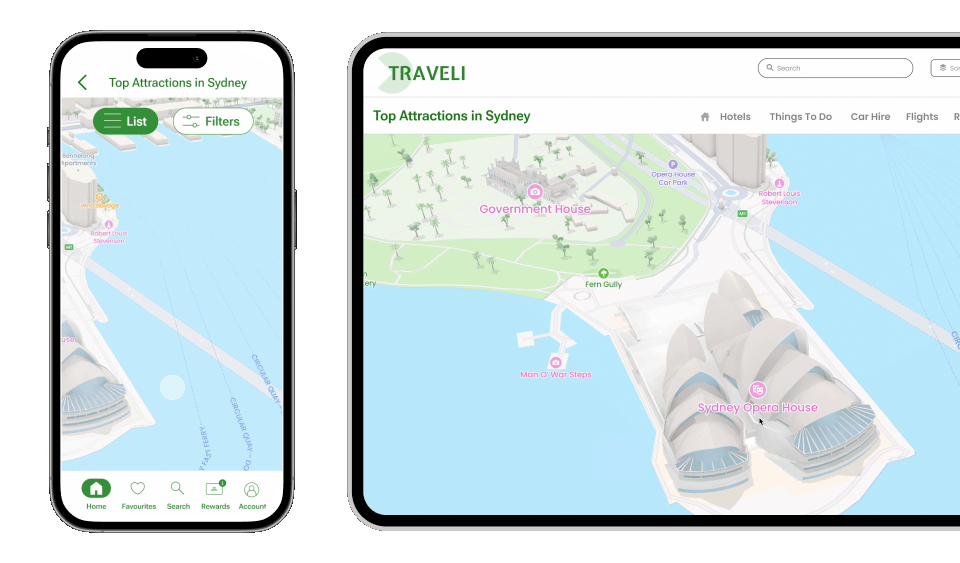
Leader in 3D rendering
Heading
Urban buildings, landmarks, and terrain come alive with 3D and dynamic lighting.
Premier performance
Heading
GL JS improves map load speed by up to 50% on average.
Delightful for developers
Heading
Ready-made basemaps, robust documentation, and 100+ code examples.
Get started quickly
Start building a web map in minutes using our quick start example.
let map = new mapboxgl.Map({
container: 'map',
center: [-122.432, 37.791],
zoom: 13.5,
style: 'mapbox://styles/mapbox/streets-v11',
accessToken: '<your_access_token>'
});
map.addControl(new mapboxgl.NavigationControl());
map.flyTo({
center: [-77.036, 38.895],
speed: 0.1
});
container: 'map',
center: [2.2940, 48.8598],
zoom: 15,
pitch:60,
accessToken:
'<your_access_token>'
});
container: 'map',
center: [2.2940, 48.8598],
zoom: 15,
pitch: 60,
accessToken: '<your_access_token>'
});
Real-time rendering meets optimal performance
Style maps at runtime with the powerful performance of the Mapbox web renderer.
Build dynamic, interactive maps
Create rich, powerful mapping experiences that change dynamically with live data. Build map features that users can interact with and that facilitate data analysis and better decision making.
Engage website visitors immediately
GL JS is engineered to render even the most detailed, feature-dense maps at 60 FPS on both desktop and mobile devices. Prioritization of resource loading and task distribution means maps load fast, transition smoothly, and leave more CPU resources available.
Elevate web map experiences
GL JS delivers innovative design, flexible controls, and superior rendering of custom data at scale.
Enhance spatial context with 3D
Mapbox weaves 3D naturally into the fabric of digital maps. Use the Mapbox Standard style, enable 3D terrain, or leverage Globe View to deliver an immersive and accurate map experience.
.png)
Control more of the map
Atmosphere properties like stars and fog improve realism. Camera and animation capabilities highlight locations from any angle. Choose from multiple map projections that gently adapt to zoom level for maximum accuracy at any scale.
Visualize large-scale data
Use heatmaps, clustering, data-driven expressions and other features to display data like never before in an interactive map. GL JS uses high-performance algorithms to render millions of features.
Save development time and resources
Mapbox GL JS supports the ways that web developers build and visualize maps, with efficient, modern development tools and highly scalable architecture.
Simplify development, augment functionality
Map features work seamlessly across web and mobile. Plus, utilize map design tools and cross-platform plugins for Directions and Search.
Streamline custom data workflows
Save time and resources when processing and hosting custom map data. Integrate custom datasets of any scale into maps quickly, affordably, and flexibly.
Upload data →Web Maps Resources
Heading
A comprehensive suite of resources for building web maps.
Getting started
Heading
Embed custom maps into a website.
Migration Guide
Heading
Resources for upgrading to GL JS v3.
Examples
Heading
Build with example code to experiment with features and get started fast.
Source Code
Heading
Read the source, submit issues, and contribute improvements to the project’s GitHub repository.
Style Spec
Heading
See all the layers, styling options, and expressions for map design.
Ready to get started?
Heading
Create an account or talk to one of our experts.