Maps for iOS and Android
SDKs for Apple and Android and support for modern tools simplify development and unify maps across devices.
Mobile maps in 3D
3D maps for cities and terrain enhance location context and raise the bar for mobile map design.
Fast rendering
The Mobile SDKs improve load time by up to 40%, with smooth transitions and offline support.
Build maps for mobile in minutes
The smoothest way to build mobile maps
Intuitively written SDKs and Mapbox styles make mobile map development a delight. Build with native SDKs for iOS and Android or use other development frameworks. It’s simple to get started building maps for your mobile applications.
Tailor maps for unique applications
Visualize parking spots, monitor a fleet of vehicles, display real estate listings, or promote nearby hiking trails. The Mobile Maps SDKs offer the flexibility and performance to meet the needs of the end user.
.png)
Engage app users immediately
Industry-leading mobile map rendering ensures maps load fast and transition smoothly, even with dense data layers and game-like graphics. Advanced profiling and debugging tools provide vital rendering performance metrics and help improve performance.
Elevate map experiences on mobile
Mapbox Mobile Maps SDKs delight users with dynamic, real-time rendering and innovative map interface options.
Enhance wayfinding and usability in 3D
Leverage the Mapbox Standard style with a 3D basemap and dynamic lighting. Use globe view for data that is continental in scale. Enable 3D terrain across your applications. The powerful mobile renderer easily handles 3D experiences that drive user engagement.
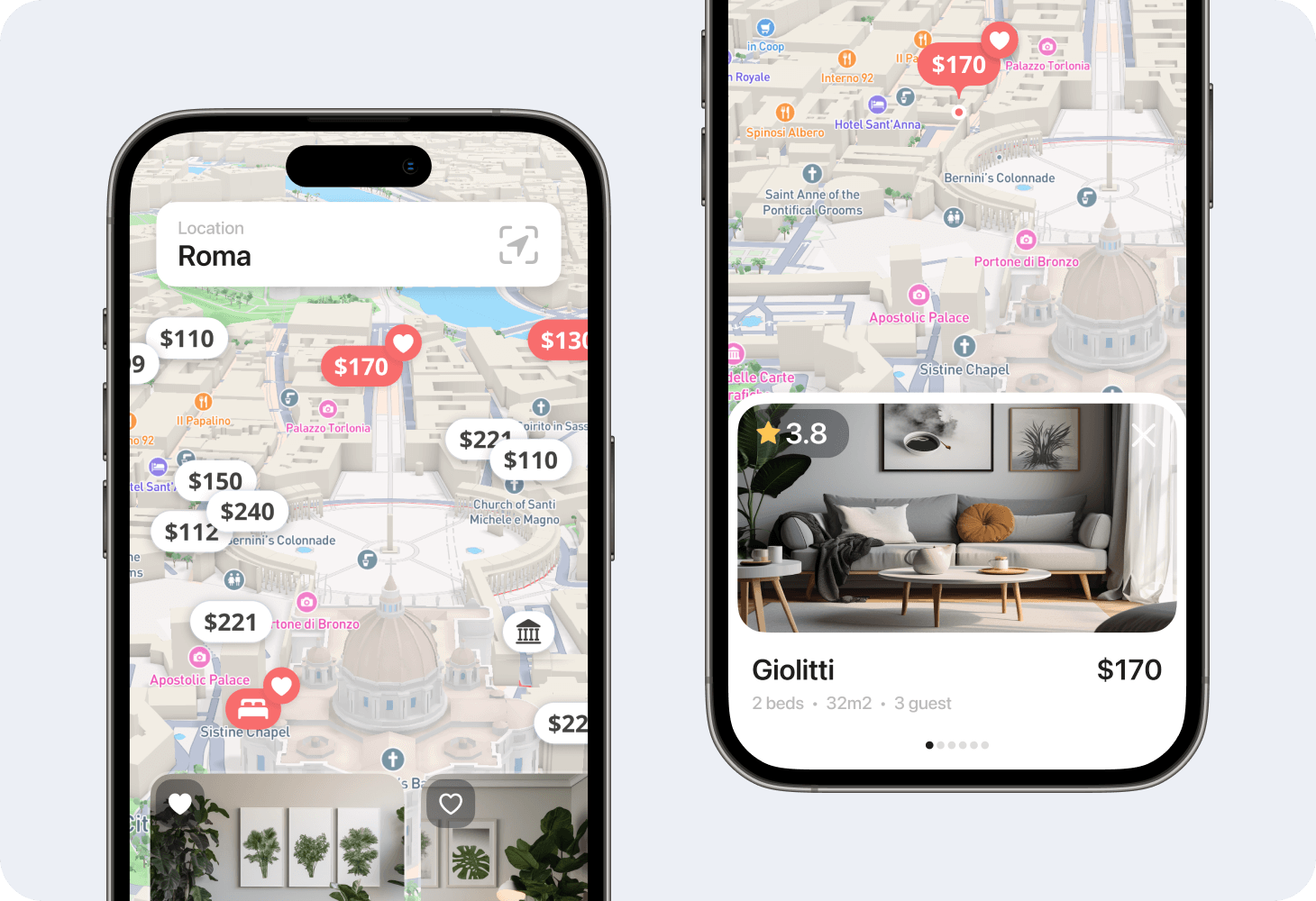
Optimize from any angle
Use flexible camera and animation capabilities to highlight any map location. Atmosphere properties like stars, haze, and fog improve realism. Integration with native platform animation systems means scenes are performant and seamless.
Solve for offline functionality
Mapbox mobile maps can provide full functionality even in low-bandwidth or offline areas. Deliver a reliable map experience, especially for premium subscribers.
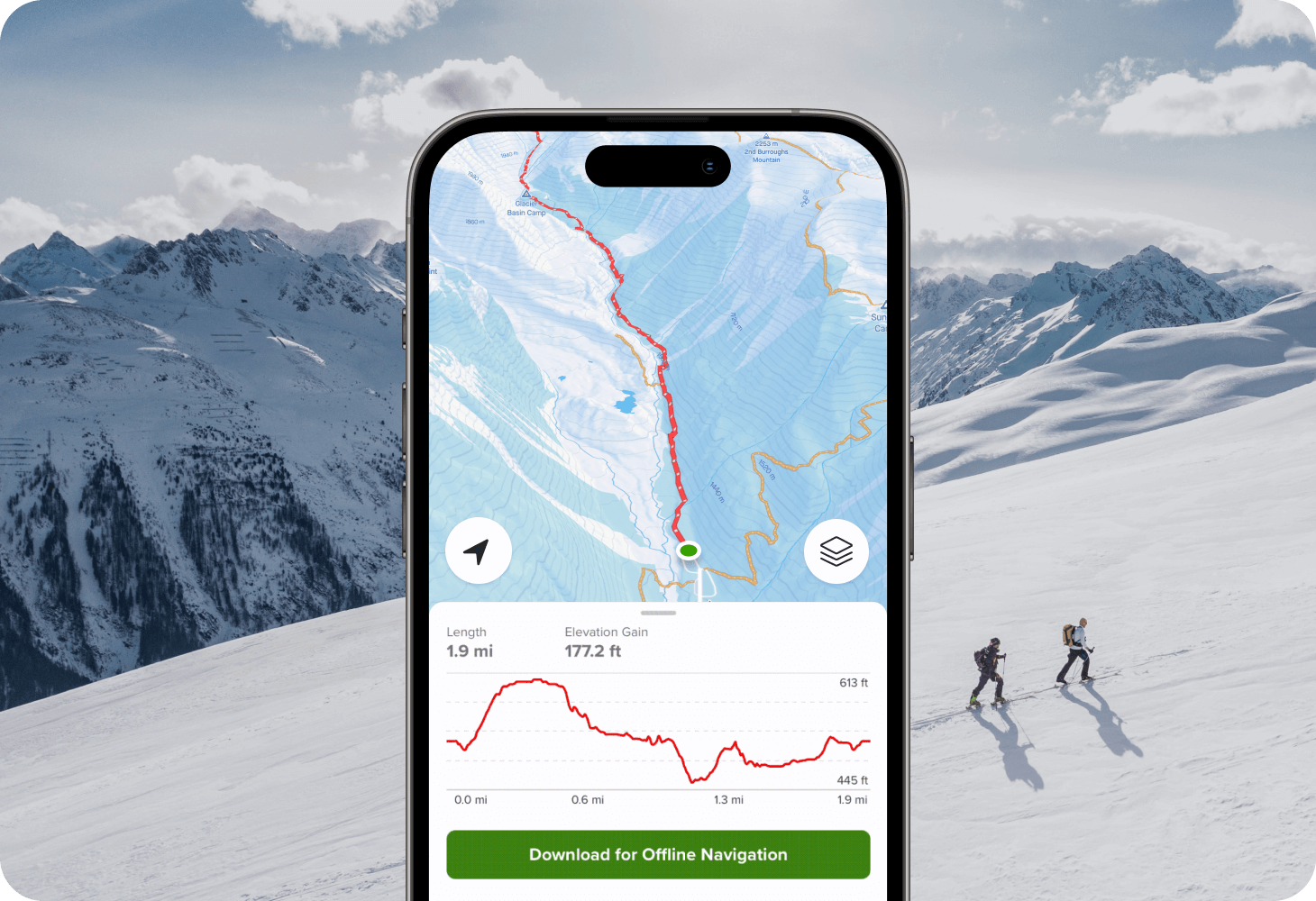
Save development time and resources
Provide developers with efficient, modern tools to build highly scalable app features.

Flexible support for industry standards
The Mobile Maps SDKs are built with support for Jetpack Compose, Swift UI, and Kotlin, as well as standards like SPM and Metal and frameworks like Flutter. Developers can work with their tools and languages of choice.
New Flutter Plugin →Streamline custom data workflows
Save time and resources when processing and hosting custom map data. Integrate custom datasets of any scale into maps quickly, affordably, and flexibly.
Upload Data →Customer stories
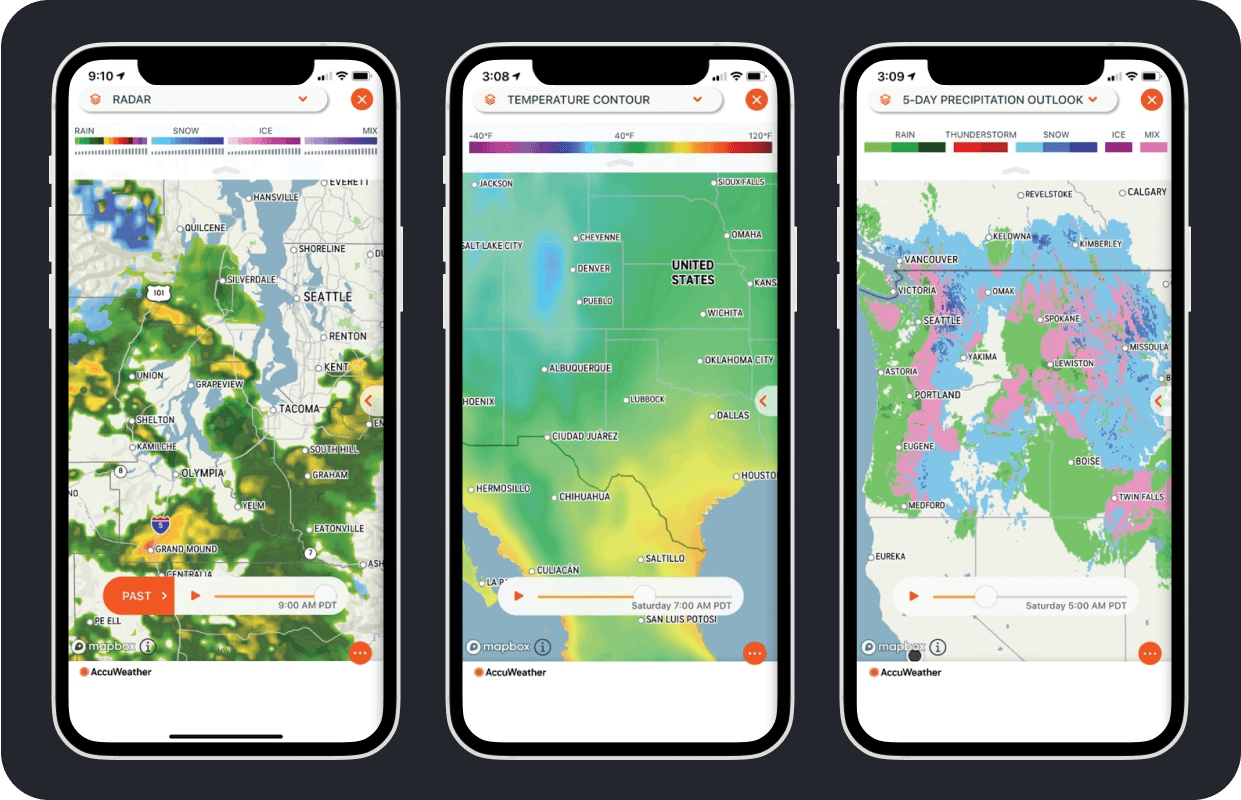
Maps are an integral part of the AccuWeather experience. The Mapbox Mobile Maps SDKs helped streamline development cycles and enabled AccuWeather to consolidate from six different mapping providers to just one.
Read showcase →

To comply with complex regulations for shared micromobility providers, Tier relies on the Mapbox Mobile Maps SDKs to efficiently load zone and vehicle data in real time.
Read showcase →

Ring users can see and share security updates and get real-time alerts based on a “neighborhood radius” of their choice — all contextualized on a map smoothly rendered by the Mapbox Mobile Maps SDKs.
Read showcase →

Seamless integration with Mapbox Mobile Maps SDKs enables AllTrails customers to access a wealth of current and meticulously detailed trail maps for hiking, biking, and camping - even in areas of low or no connectivity.
Read showcase →