When we build web applications at Mapbox, we often turn to React and Mapbox GL JS. The libraries work powerfully in combination, and I’d like to share some techniques for connecting the two.
The power of React lies in being an abstraction on top of the DOM. What’s shown to the user when a component calls render() is conveniently managed internally allowing an implementor to work on higher level tasks like how an interface behaves.
What can be confusing is connecting React with other libraries that manipulate the DOM and manage state (like Mapbox GL JS). How do you communicate between both effectively? It’s common to turn to component wrappers that provide a level of abstraction that hides this confusion away. That technique is effective for enforcing a standardized set of rules passed as props across your application. An example may be a modal component. The wrapper permits customization like size or title, and the technical details that should always be the same (event handling of key bindings or accessibility) are tucked away in the lower level modal component.
So it’s natural to look for a <Mapbox /> component within the React ecosystem (see react-map-gl or react-mapbox-gl as example). The goals however of a Modal component are small and applying this same technique to a feature rich library is a high tradeoff. If you are missing functionality, it’s up to the maintainer to expand support through additional props.
Thankfully Mapbox GL JS works well without a wrapper abstraction. It’s pretty easy for third-party libraries to work alongside React!
The entry point to initialize a map is through a single element provided in the return statement of your render function. Here’s a quick example:
JSX creates the div container and the map is continuously updated through lifecycle methods. The key is that ref attribute. The map is initialized within componentDidMount and its container value is set as the assignment of this.mapContainer which is React’s way of providing direct access to the DOM node.
Let’s explore some more in-depth examples:
Basic example
In this example, React passes position data as a state to the map. That state updates by listening to the move event and a container outside the map displays those values. This could also be prop data passed from a higher level component but in the interest of containing the entire app as one component I’ve used state.
Reactive tooltip example
The details to note are:
- Data found in vector tiles is collected under the mouse cursor using queryRenderedFeatures
- A mapboxgl.Marker instance is used to display the collected data on the map (Mapbox GL JS controls its position on the map) but the contents of the marker is React powered using ReactDOM.render.
Fetching map data like this isn’t exclusive to tiles directly from Mapbox. Any data layers you add using addSource can apply this same technique. This is a powerful way to store a lot of data and request it on an as-needed basis.
Data overlay example
Here GeoJSON is provided by the app and added as a source to the map. Click handlers instruct how the data should be re-styled which the map responds to when componentDidUpdate is called.
For more complex applications where numerous components require knowledge of the same state of data we use Redux. I’ve provided a version of this example using Redux that models a similar architecture to Studio. To learn more how we use Redux in Mapbox Studio, check out, Redux for state management in large web apps by David Clark.
I hope this helps as a primer on using Mapbox GL JS alongside React and provides some context for how we achieve a few different concepts here at Mapbox. To suggest improvements to any of these demos or to learn more visit https://github.com/mapbox/mapbox-react-examples/
For more reading check out Tom MacWright’s post on Mapbox GL JS in a reactive application. He describes how we applied many of the render concepts from React directly into Mapbox GL JS to quickly apply style updates. If this stuff interests you, check out our job openings in engineering. We’re hiring!
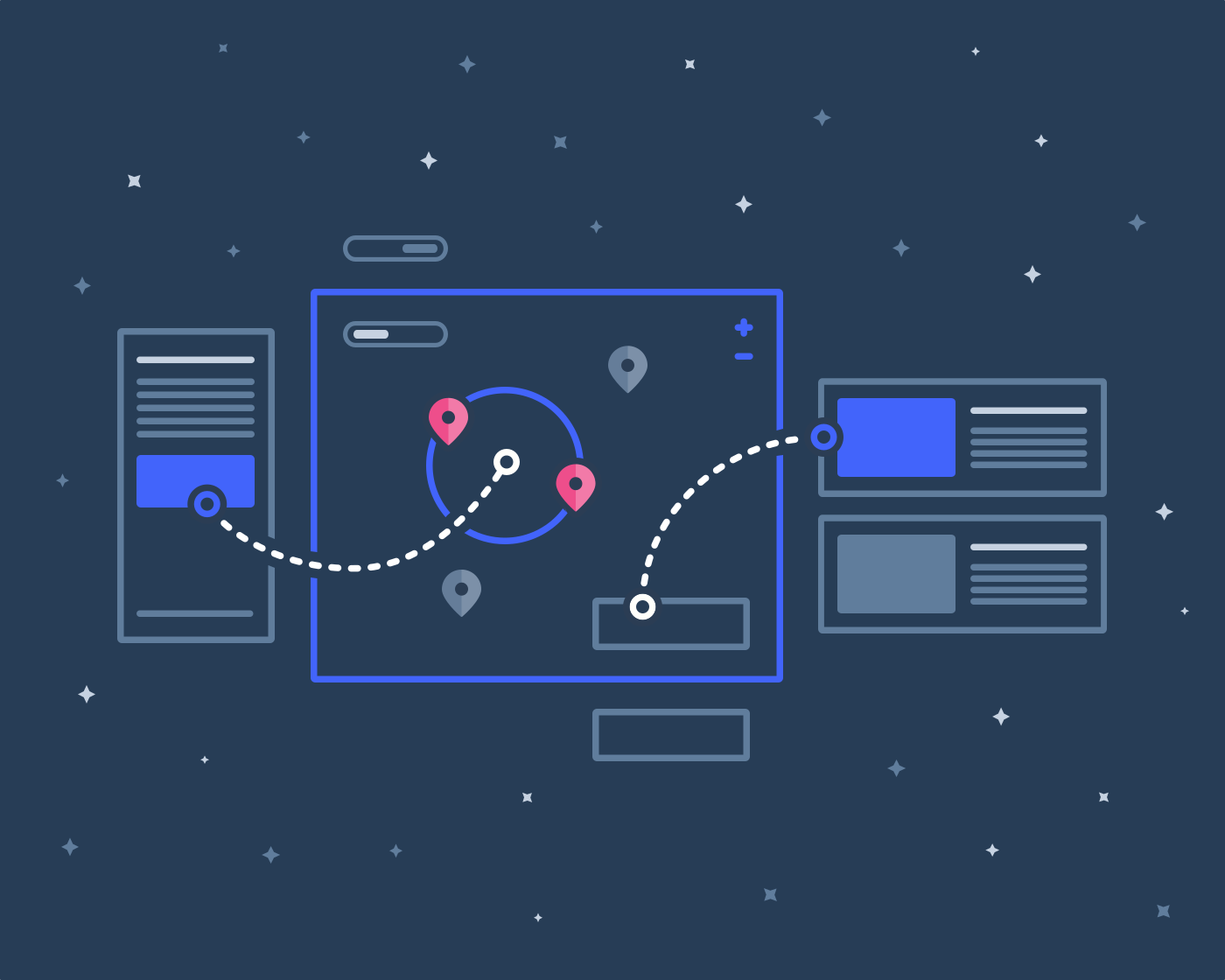